Simple Scene
The simple scene composes of a skybox and a box.
1) When depth test is enabled, draw Box, and then Skybox, the scene looks good:
/// before render loop, enable depth test
glEnable(GL_DEPTH_TEST);
/// in render loop, draw cube and then skybox
// draw cube first
shader.use();
glm::mat4 model = glm::mat4(1.0f);
glm::mat4 view = camera.GetViewMatrix();
glm::mat4 projection = glm::perspective(glm::radians(camera.Zoom), (float)SCR_WIDTH / (float)SCR_HEIGHT, 0.1f, 100.0f);
shader.setMat4("model", model);
shader.setMat4("view", view);
shader.setMat4("projection", projection);
glBindVertexArray(cubeVAO);
glActiveTexture(GL_TEXTURE0);
glBindTexture(GL_TEXTURE_2D, cubeTexture);
glDrawArrays(GL_TRIANGLES, 0, 36);
glBindVertexArray(0);
// draw skybox at last
glDepthFunc(GL_LEQUAL); // change depth function so depth test passes when values are equal to depth buffers's content
skyboxShader.use();
model = glm::mat4(1.0f);
projection = glm::perspective(glm::radians(camera.Zoom), (float)SCR_WIDTH / (float)SCR_HEIGHT, 0.1f, 100.0f);
view = glm::mat4(glm::mat3(camera.GetViewMatrix())); // remove translation from the view matrix
skyboxShader.setMat4("view", view);
skyboxShader.setMat4("projection", projection);
glBindVertexArray(skyboxVAO);
glActiveTexture(GL_TEXTURE0);
glBindTexture(GL_TEXTURE_CUBE_MAP, cubemapTexture);
glDrawArrays(GL_TRIANGLES, 0, 36);
glBindVertexArray(0);
glDepthFunc(GL_LESS); // set depth function back to default
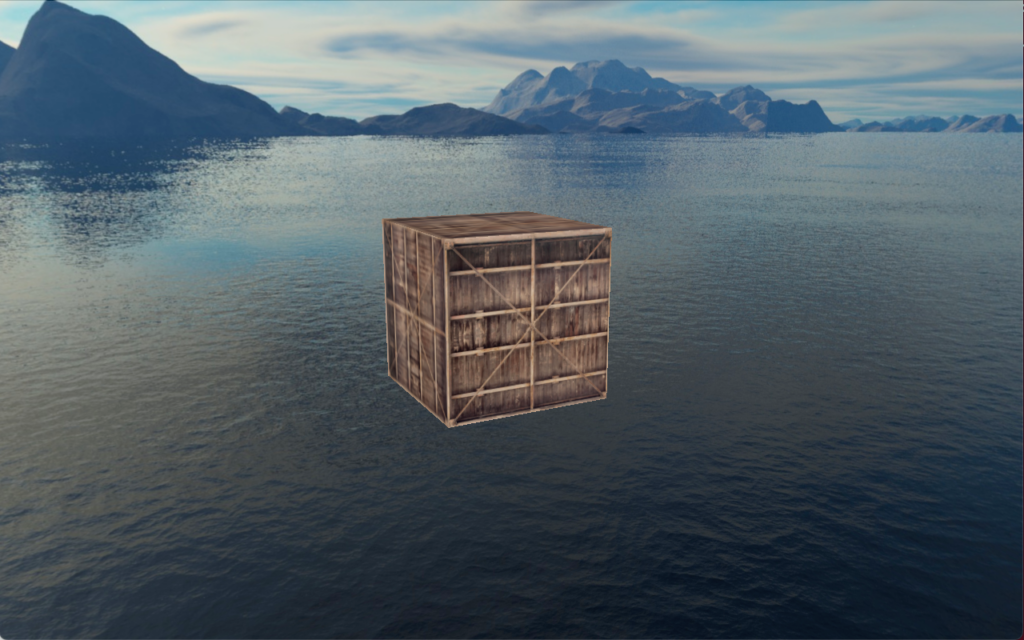
2) When depth test is disabled, draw Box, and then Skybox, Box is gone, due to draw override, the later drawn Skybox overrides the previously drawn Cube, just simply a painter’s algorithm.
/// before render loop, disable depth test
//glEnable(GL_DEPTH_TEST);
/// in render loop, draw cube and then skybox
// code is the same with above
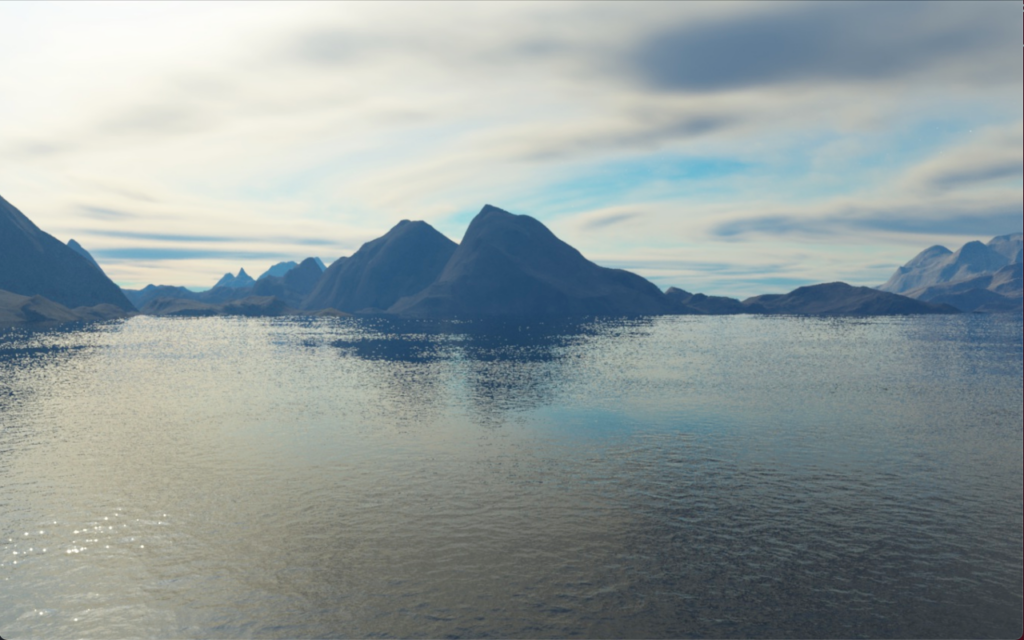
3) When depth test is disabled, how about draw Skybox, and then Box? Box is drawn now, but scene looks not right, look at the box, due to no depth test, the cube face relationship is incorrect in terms of depth.
/// before render loop, disable depth test
//glEnable(GL_DEPTH_TEST);
/// in render loop, draw cube and then skybox
// draw skybox first
glDepthFunc(GL_LEQUAL); // change depth function so depth test passes when values are equal to depth buffers's content
skyboxShader.use();
glm::mat4 model = glm::mat4(1.0f);
glm::mat4 projection = glm::perspective(glm::radians(camera.Zoom), (float)SCR_WIDTH / (float)SCR_HEIGHT, 0.1f, 100.0f);
glm::mat4 view = glm::mat4(glm::mat3(camera.GetViewMatrix())); // remove translation from the view matrix
skyboxShader.setMat4("view", view);
skyboxShader.setMat4("projection", projection);
glBindVertexArray(skyboxVAO);
glActiveTexture(GL_TEXTURE0);
glBindTexture(GL_TEXTURE_CUBE_MAP, cubemapTexture);
glDrawArrays(GL_TRIANGLES, 0, 36);
glBindVertexArray(0);
glDepthFunc(GL_LESS); // set depth function back to default
// draw cube at last
shader.use();
model = glm::mat4(1.0f);
view = camera.GetViewMatrix();
projection = glm::perspective(glm::radians(camera.Zoom), (float)SCR_WIDTH / (float)SCR_HEIGHT, 0.1f, 100.0f);
shader.setMat4("model", model);
shader.setMat4("view", view);
shader.setMat4("projection", projection);
glBindVertexArray(cubeVAO);
glActiveTexture(GL_TEXTURE0);
glBindTexture(GL_TEXTURE_2D, cubeTexture);
glDrawArrays(GL_TRIANGLES, 0, 36);
glBindVertexArray(0);
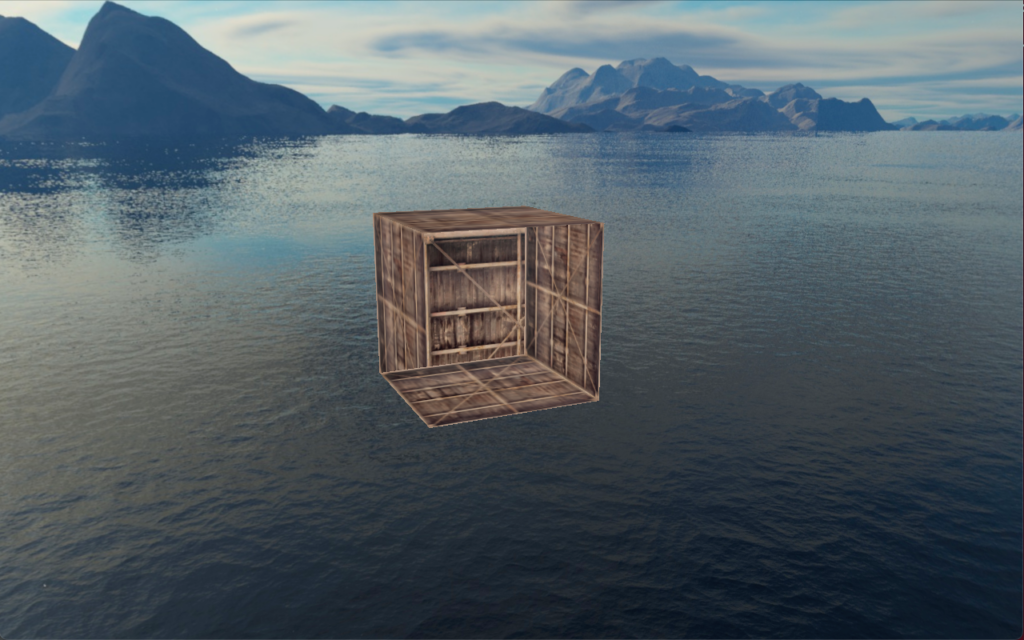
4) When depth test is enabled, how about draw Skybox, and then Box? The scene also looks good.
/// before render loop, enable depth test
glEnable(GL_DEPTH_TEST);
/// in render loop, draw cube and then skybox
// code is the same with above
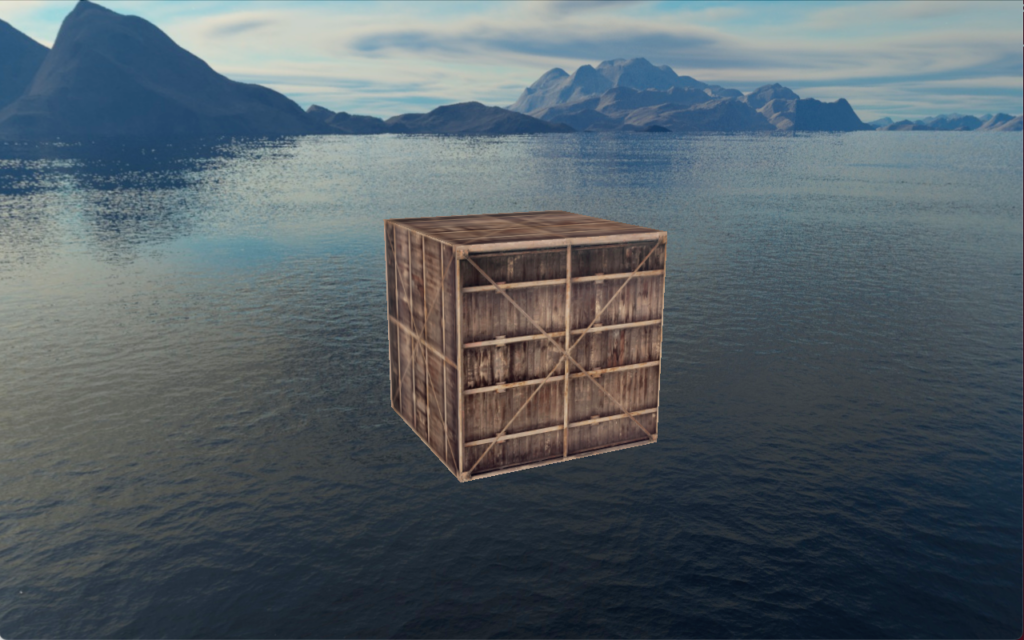
Conclusion
For such a simple scene, only contains a skybox and a box, when depth test is enabled, draw sequence doesn’t matter as long as the box is in front of the skybox in world space.
When depth test disabled, draw sequence matters, the later drawn pixels will override the previously drawn pixels, although box can be drawn when it’s drawn later, the box faces’ occlusion is wrong, so depth test is necessary in this case.
For the complete code, checkout the cubemap_skybox chapter on learnopengl.com.